JavaScript fundementals: Bind, Call and Apply
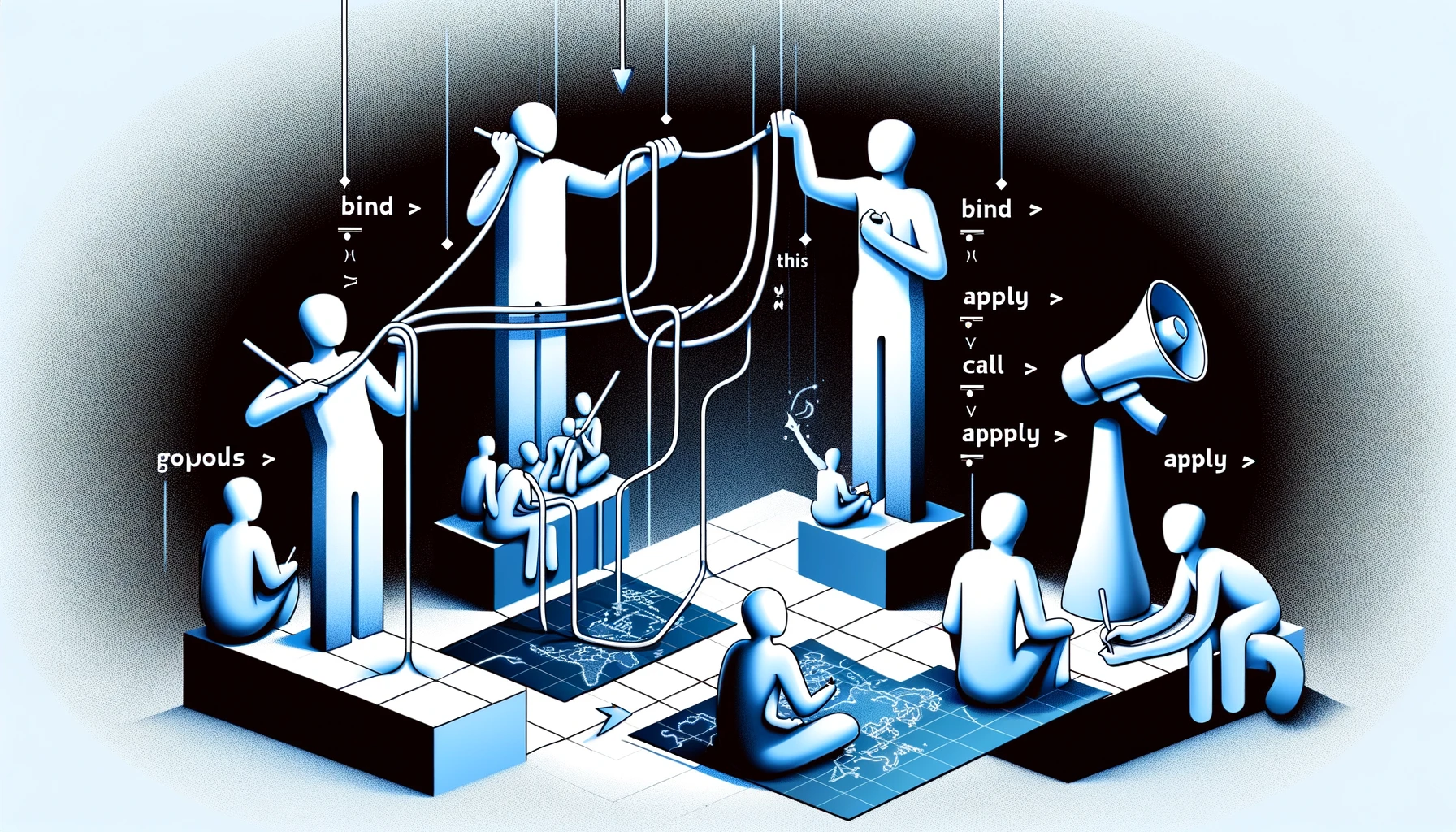
Understanding bind
, call
, and apply
is crucial for effective JavaScript programming, especially when dealing with the context (this
) or when you need to control the execution context of a function. These three methods allow you to explicitly define what this
should refer to when a function is executed, but they do so in slightly different ways.
call
The call
method immediately invokes the function with a specified this
value and arguments provided individually.
- Use Case: When you need to invoke a function and you know the arguments that need to be passed to the function ahead of time.
- Benefits: Allows for a function belonging to one object to be assigned and called for a different object.
- Limitation: Arguments have to be known at the time of calling and cannot be dynamically prepared as an array.
function greet(message, name) {
console.log(`${message}, ${name}. This message is from a ${this.type} messenger.`);
}
const emailMessenger = { type: 'email' };
// Using call to invoke greet with emailMessenger as `this`
greet.call(emailMessenger, 'Hello', 'Alice');
Here, call
is used to invoke the greet
function immediately, specifying the this
context as emailMessenger
, and passing the arguments 'Hello'
and 'Alice'
individually.
Analogy: Think of a remote-controlled toy car. The call
method is like pressing a button on the remote control to make the car move immediately. The button you press determines the direction (analogous to the function you’re invoking) and the remote control frequency determines which car responds (analogous to the this
context you’re setting). You decide the speed and direction by the way you press the button (analogous to the arguments you pass one by one).
function driveCar(direction, speed) {
console.log(`The ${this.color} car is moving ${direction} at ${speed} mph.`);
}
const redCar = { color: 'red' };
// Using the call method:
driveCar.call(redCar, 'forward', 50);
Explanation:
- We have a function
driveCar
that accepts a direction and speed. This function represents pressing a button on a remote control to make the car move. - The object
redCar
represents a specific car that the remote can control. - When we use
call
, the first argument (redCar
) sets thethis
context for the function. This is analogous to determining which car responds to the remote. - The subsequent arguments (
'forward'
and50
) are passed to thedriveCar
function, just like determining the direction and speed of the car.
Test your knoweldge:
Call Method Quiz
apply
The apply
method is similar to call
, but instead of taking arguments individually, it takes them as an array.
- Use Case: When the arguments to the function are already in an array or when you don’t know the number of arguments that will be passed to the function.
- Benefits: Useful for functions where the number of arguments is not fixed or when arguments are dynamically determined.
- Limitation: Less intuitive when dealing with a fixed number of arguments.
function updateInventory(items) {
console.log(`Adding items: ${items.join(', ')} to the inventory.`);
}
const inventoryUpdateList = ['Apples', 'Oranges', 'Bananas'];
// Using apply to invoke updateInventory with an array of items
updateInventory.apply(null, [inventoryUpdateList]);
In this scenario, apply
enables the updateInventory
function to be called with a dynamic list of items to add to the inventory.
Analogy: Imagine a paintbrush (the function) and a paint palette with multiple colors (the array of arguments). Using the apply
method is like dipping the paintbrush into multiple colors at once to create a blended effect on canvas. The canvas represents the this
context, the action of painting is the function execution, and the particular blend of colors is determined by the array of arguments.
function paintCanvas(colors) {
console.log(`Painting with ${colors.join(', ')} on the ${this.size} canvas.`);
}
const largeCanvas = { size: 'large' };
const colorPalette = ['red', 'blue', 'yellow'];
// Using the apply method:
paintCanvas.apply(largeCanvas, [colorPalette]);
Explanation:
- We have a function
paintCanvas
which accepts an array of colors. This represents the paintbrush dipping into different colors. - The
largeCanvas
object represents the canvas on which we’re painting. - When using
apply
, the first argument sets thethis
context (thelargeCanvas
in this case). - The second argument is an array of parameters to pass to the function. This is like picking multiple colors from a palette to use at once.
Test your knoweldge:
Apply Method Quiz
bind
The bind
method creates a new function that, when called, has its this
keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
- Use Case: When you need to pass a function as a callback but ensure it still executes in the correct
this
context. - Benefits: Allows for the pre-configuration of the function with specific arguments, making it ready to use at a later time.
- Limitation: The bound function needs to be called separately, making it less suitable for immediate invocation.
function logActivity(activity, time) {
console.log(`Activity: ${activity} at ${time} hours. [${this.user}]`);
}
const userActivityLogger = { user: 'JohnDoe' };
// Binding logActivity to userActivityLogger
const logJohnsActivity = logActivity.bind(userActivityLogger, 'Running');
// Using the bound function at a later time
logJohnsActivity('10:00');
This demonstrates using bind
to preconfigure a function, logActivity
, to log activities for a specific user, making it possible to reuse this setup without repeating the context and initial argument setup.
Analogy: Think of creating a custom preset on a coffee machine. You have a general coffee machine (the function) and you can set a preset for your favorite type of coffee (like a latte with a specific amount of milk and coffee). The bind
method is like setting that preset. Once the preset is saved (analogous to the bind
function), anyone (or any event in the future) can press that preset button and get the exact type of coffee you specified without needing to select the individual settings again. The specific coffee machine you’ve set this preset on is analogous to the this
context, and the specific settings (like amount of milk, coffee strength, etc.) are analogous to the arguments.
Remember, while analogies can provide a more intuitive grasp of concepts, they also have their limits and might not capture every nuance of the actual concepts.
function brewCoffee(milkAmount, coffeeStrength) {
console.log(`Brewing a ${this.type} coffee with ${milkAmount} ml of milk and ${coffeeStrength} strength.`);
}
const latteMachine = { type: 'latte' };
const makeMyFavoriteCoffee = brewCoffee.bind(latteMachine, 150, 'medium');
// Using the bound function:
makeMyFavoriteCoffee();
Explanation:
- The
brewCoffee
function represents the action of brewing a type of coffee with specific milk and strength settings. - The
latteMachine
object represents the specific coffee machine preset. - The
bind
method creates a new function,makeMyFavoriteCoffee
, which is a version ofbrewCoffee
pre-configured with specific arguments. This is like setting a custom preset on the coffee machine. - Later, when we invoke
makeMyFavoriteCoffee
, it remembers both the context (latteMachine
) and the preset parameters (150
for milk and'medium'
for coffee strength).
Test your knoweldge:
Bind Method Quiz
Closing Thoughts
The bind, call, and apply methods in JavaScript serve as essential tools for precise control over the execution context of functions. Each method offers a unique approach to manipulating the this keyword, thereby providing developers with the flexibility needed to handle various programming scenarios efficiently. Through engaging analogies and practical examples, we’ve explored how call enables immediate function invocation with individually specified arguments, apply facilitates function calls with arguments passed as an array, and bind allows for the creation of pre-configured functions for later use. Understanding and effectively applying these methods can significantly enhance code quality and functionality, especially in complex environments where dynamic context management is key. This exploration underscores the importance of mastering these methods for anyone looking to deepen their JavaScript expertise and develop more sophisticated, robust web applications.